What is the difference between ways to point in arrays?
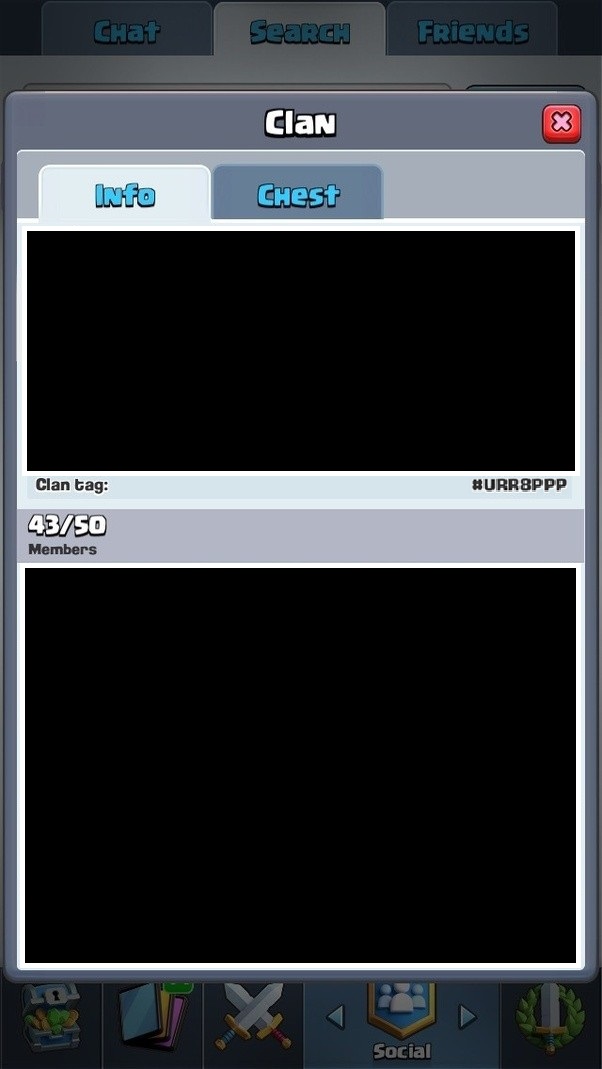

What is the difference between ways to point in arrays?
Assume that I define x as array like this: int x[30]=2,4,8,9,6,3,5,4,7,8,9,6,3,2,
and A
as a pointer to an int
like this: int *A
.
int x[30]=2,4,8,9,6,3,5,4,7,8,9,6,3,2,
A
int
int *A
Now I want to know what the difference is between these:
int *A=x[5]
int *A=x[5]
int *A ; A=&x[5]
int *A ; A=&x[5]
int *A=x+5
int *A=x+5
int *A ; *A=x+5
int *A ; *A=x+5
int *A ; A=*(x+5)
int *A ; A=*(x+5)
Why do number 1, 4 and 5 give an error?
Number 4 gives an error although number 3 doesn't. Why are these not equal?
1 Answer
1
In number 1, you're creating a variable A
which is a pointer to an int
, and x[5]
is an int
, not a pointer. So you're giving a pointer an int
value, which produces an error.
A
int
x[5]
int
int
In number 2, you create a pointer to an int
and then make it point to the variable x[5]
, since &x[5]
is the address of x[5]
. That will compile just fine and the way you wrote it won't produce any error, however creating a pointer without any initial value is not recommended. When you create A
without initializing it, it will take the value that was already in the memory which can be any value. Therefore, if you use the variable A
before giving it an error, your program will crash. Instead, it's recommended to do:
int
x[5]
&x[5]
x[5]
A
A
int *A = NULL;
A = &x[5];
or simply:
int *A= &x[5];
In number 3, you're creating a pointer to an int
and you're assigning it the value x + 5
. This is OK since x
is in fact a pointer to x[0]
(x
and &x[0]
do exactly the same thing). So x + 5
is a pointer to the value that is 5 memory spaces after x[0]
, which is x[5]
. In fact, when the compiler sees x[5]
, it converts it to *(x + 5)
.
int
x + 5
x
x[0]
x
&x[0]
x + 5
x[0]
x[5]
x[5]
*(x + 5)
In number 4, A
is a pointer to an int
, so *A
is the value which A
is pointing to, which is an int
. In you're code, *A = x + 5
is wrong for two reasons: For the first reason, the code won't compile since x + 5
is a pointer and *A
is an int
, so you're assigning a pointer value to an int
, which isn't valid. But there is another error in there. Imaging it compiled for some reason. Then, at runtime, what it does, is that it takes the memory space that A
is pointing to and gives it the value x + 5
. The problem is that A
isn't initialized so it's very likely that A
points to a variable used by another program. To prevent this from doing any damage, your OS will make the program crash. If you're "lucky", A
will point to a variable in your program so it will assign a new value to a variable in your program without you knowing which variable it is, so your program will continue running but won't work correctly.
A
int
*A
A
int
*A = x + 5
x + 5
*A
int
int
A
x + 5
A
A
A
In number 5, A
is a pointer to an int
and you're assigning it the value *(x + 5)
. As I explained earlier, *(x + 5)
is exactly the same thing as x[5]
, which is an int
. So you're assigning an int
value to a pointer, which is not valid. Note that if for some reason you want to assign an int
value to a pointer, you could do A = (int*)(*(x + 5))
, which would compile properly (but the program would probably crash at runtime). Then A
would be a pointer pointing to the memory space 3
. In the same way, you can also do x[5] = (int)A
, which stores the address of the variable that A
is pointing to as an int
in x[5]
. However, I've never seen any situation where these kinds of things would be useful in practice.
A
int
*(x + 5)
*(x + 5)
x[5]
int
int
int
A = (int*)(*(x + 5))
A
3
x[5] = (int)A
A
int
x[5]
...since x is in fact a pointer to x[0]...
x
sizeof
x + 5
x + 5
x
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
Re:
...since x is in fact a pointer to x[0]...
. You need to be careful when explaining arrays and pointers.x
is not a pointer, it's an array. But when it appears in an expression (other than argument ofsizeof
; in our casex + 5
is the expression), it gets converted to a pointer to the first element of the array. And then we deal with pointer arithmetic. Adding 5 to a pointer advances the pointer by 5 elements, sox + 5
ends up pointing to element 5 of arrayx
.– Alexey Frunze
Dec 17 '16 at 21:59