how to parse json into struct in swift
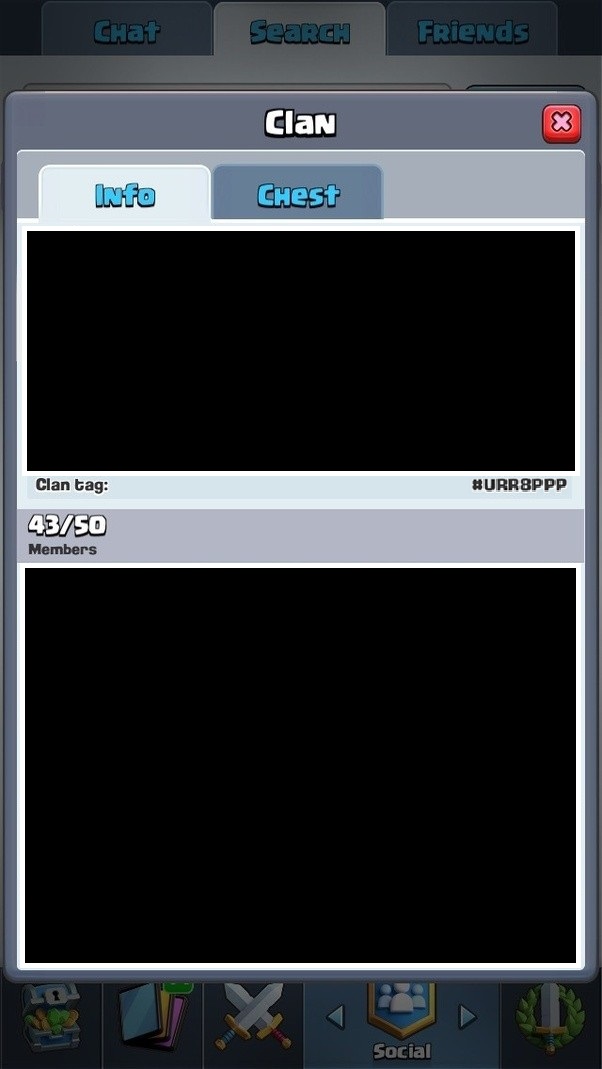

how to parse json into struct in swift
I have struct like this:
struct Company
let name:String
let id:Int
I want to parse from JSON a set of Companies.
Can you please help me how can I do that in Swift?
3 Answers
3
Unfortunately JSON parsing is not very easy in swift.
You should use the NSJSONSerialization class to do it.
There are plenty of examples here to look at.
In Swift3, It's possible to convert dictionary direct to struct use MappingAce
struct Company: Mapping
let name:String
let id:Int
let companyInfo: [String : Any] = ["name" : "MappingAce", "id" : 1]
let company = Company(fromDic: companyInfo)
print(company.name)//"MappingAce"
print(company.id) // 1
This is for future folks. In Swift 4 there is a very nice solution with JSONDecoder
.
JSONDecoder
With Alamofire code will look like this -
Alamofire.request(Router.login(parameters: parameters)).responseJSON
response in
switch response.result
case .success(_):
let decoder = JSONDecoder()
guard let _ = response.data else
return
do
let loginDetails = try decoder.decode(LoginDetails.self, from: response.data!)
// get your details from LoginDetails struct
catch let err
print(err)
case .failure(let error):
print(error)
https://developer.apple.com/documentation/foundation/jsondecoder
https://medium.com/xcblog/painless-json-parsing-with-swift-codable-2c0beaeb21c1
Hope this helps!!!
guard let _
is very bad syntax if you actually need the bound variable later.– vadian
56 mins ago
guard let _
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
You can use SwiftyJSON, github.com/SwiftyJSON/SwiftyJSON
– vzamanillo
Dec 11 '14 at 11:25