Javascript ES6/ES5 find in array and change
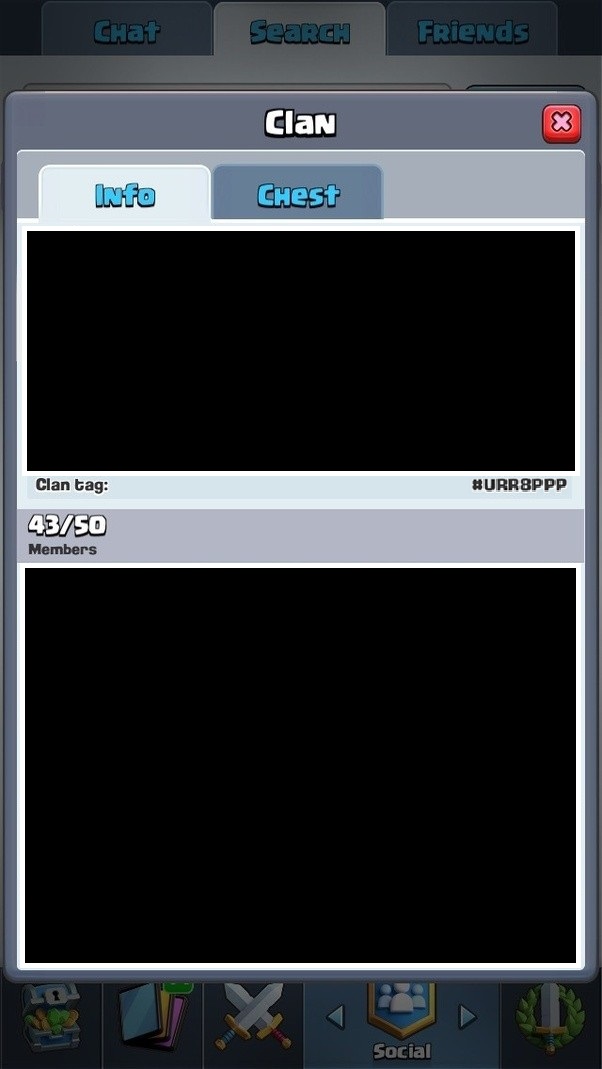

Javascript ES6/ES5 find in array and change
I have an array of objects.
I want to find by some field, and then to change it:
var item = ...
var items = [id:2, id:2, id:2];
var foundItem = items.find(x => x.id == item.id);
foundItem = item;
I want it to change the original object. How? (I dont care if it will be in lodash too)
3 Answers
3
You can use findIndex to find the index in the array of the object and replace it as required:
var item = ...
var items = [id:2, id:2, id:2];
var foundIndex = items.findIndex(x => x.id == item.id);
items[foundIndex] = item;
This assumes unique IDs. If your IDs are duplicated (as in your example), it's probably better if you use forEach:
items.forEach((element, index) =>
if(element.id === item.id)
items[index] = item;
);
items.map(x => x.id == item.id ? item : x)
@georg That would return a new array though.
– CodingIntrigue
Feb 4 '16 at 16:33
The => function won't work in IE11. Recently bitten by this.
– Lewis Cianci
Jan 23 at 23:44
may be it would be better to use
let
keyword instead of var
– Inus Saha
Feb 23 at 7:28
let
var
Just FYI, this does not work in some versions of phantomJS
– Sid
Mar 6 at 16:25
My best approach is:
var item = ...
var items = [id:2, id:2, id:2];
items[items.findIndex(el => el.id === item.id)] = item;
Reference for findIndex
And in case you don't want to replace with new object, but instead to copy the fields of item
, you can use Object.assign
:
item
Object.assign
Object.assign(items[items.findIndex(el => el.id === item.id)], item)
Object.assign(items[items.findIndex(el => el.id === item.id)], item)
as an alternative with .map()
:
.map()
Object.assign(items, items.map(el=> el.id === item.id? item : el))
Object.assign(items, items.map(el=> el.id === item.id? item : el))
Please link to an English page if the original question was in English. Also, this example assumes the object is always found.
– raarts
Jul 3 '17 at 10:45
You can always wrap it in a try catch expression then... right?
– Soldeplata Saketos
Jul 4 '17 at 11:11
And being completely literal to the posts' question, he wants to edit an element in an array. He doesn't want to know if it exists or not, so we assume he already did that before.
– Soldeplata Saketos
May 11 at 12:58
An other approach is to use splice.
In case you're working with reactive frameworks, it will update the "view", your array "knowing" you've updated him.
Answer :
var item = ...
var items = [id:2, id:2, id:2];
let foundIndex = items.findIndex(element => element.id === item.id)
items.splice(foundIndex, 1, item)
And in case you want to only change a value of an item, you can use find function :
// Retrieve item and assign ref to updatedItem
let updatedItem = items.find((element) => return element.id === item.id )
// Modify object property
updatedItem.aProp = ds.aProp
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
or
items.map(x => x.id == item.id ? item : x)
...– georg
Feb 4 '16 at 16:33