ASP.NET w/ Identity: Entity Framework throws System.Data.Entity.Core.UpdateException on SaveChanges
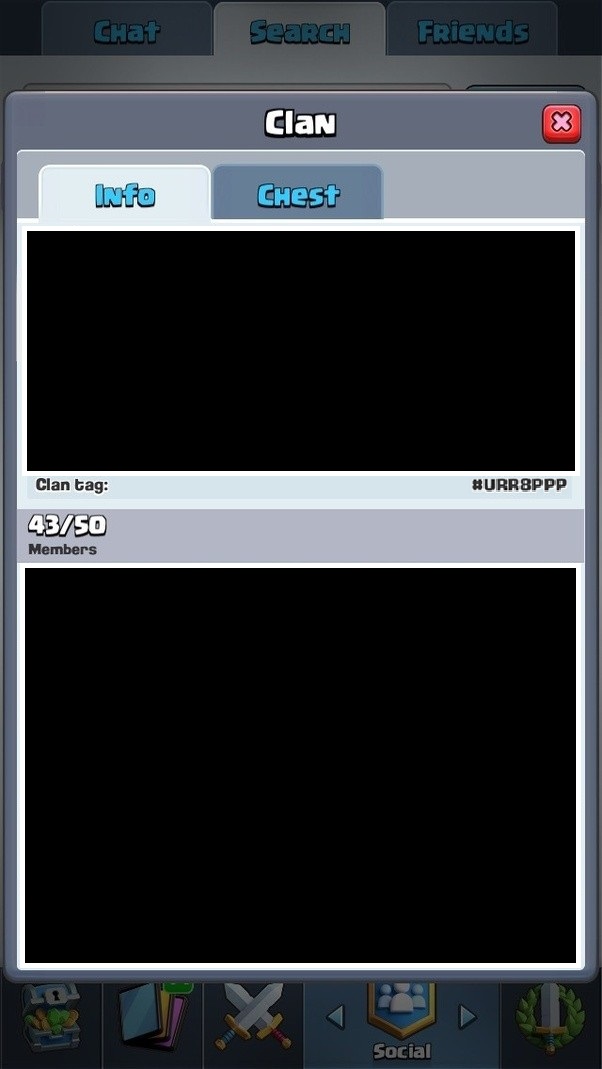

ASP.NET w/ Identity: Entity Framework throws System.Data.Entity.Core.UpdateException on SaveChanges
I am trying to build an ASP.NET application which should facilitate carpooling.
For it, I use the Identity Framework to manage accounts and Entity Framework as ORM. I use the ApplicationDbContext of Identity for my models too, so I won't have problems with the code-first migrations and because I need a one-to-one relation between a UserProfile object and the Identity's ApplicationUser.
Here are my models:
public class UserProfile
[Key]
[DatabaseGenerated(DatabaseGeneratedOption.Identity)]
public int Id get; set;
public string Name get; set;
public string FirstName get; set;
public string Email get; set;
public string UserName get; set;
public virtual List<Trip> Trips get; set; = new List<Trip>();
public virtual List<Meeting> Meetings get; set; = new List<Meeting>();
public class Trip
[Key]
[DatabaseGenerated(DatabaseGeneratedOption.Identity)]
public int Id get; set;
[Required]
public virtual TripCheckpoint Departure get; set;
[Required]
public virtual TripCheckpoint Destination get; set;
[Required]
public int Seats get; set;
[NotMapped]
public int AvailableSeats => Seats - Passengers.Count;
[Required]
public virtual UserProfile Organizer get; set;
public double Price get; set; = 0.0;
public virtual List<UserProfile> Passengers get; = new List<UserProfile>();
public virtual DateTime Date get; set;
public class TripCheckpoint
[Key]
[DatabaseGenerated(DatabaseGeneratedOption.Identity)]
public int CheckPointId get; set;
[Required]
public string Place get; set;
[Required]
public TimeSpan Hour get; set;
public class Meeting
[Key]
[DatabaseGenerated(DatabaseGeneratedOption.Identity)]
public int Id get; set;
public DateTime When get; set;
public string Where get; set;
Here is my ApplicationDbContext:
public class ApplicationDbContext : IdentityDbContext<ApplicationUser>
public DbSet<UserProfile> Profiles get; set;
public DbSet<Trip> Trips get; set;
public DbSet<TripCheckpoint> Checkpoints get; set;
public DbSet<Meeting> Meetings get; set;
public ApplicationDbContext()
: base("helmoCars", throwIfV1Schema:false)
public static ApplicationDbContext Create()
return new ApplicationDbContext();
protected override void OnModelCreating(DbModelBuilder dbBuilder)
base.OnModelCreating(dbBuilder);
dbBuilder.Entity<ApplicationUser>().HasRequired(u => u.Profile).WithRequiredPrincipal().Map(m => m.MapKey("AppUser_Id"));
dbBuilder.Entity<Trip>().HasMany(t => t.Passengers).WithMany().Map(m =>
m.ToTable("Trip_Passengers");
m.MapLeftKey("Trip_Id");
m.MapRightKey("UserProfile_Id");
);
dbBuilder.Entity<Trip>().HasRequired(t => t.Organizer).WithMany(u => u.Trips).Map(m => m.MapKey("Trip_Organizer"))
.WillCascadeOnDelete(false);
dbBuilder.Entity<Trip>().HasRequired(t => t.Departure).WithRequiredDependent().Map(m => m.MapKey("Trip_Departure")).WillCascadeOnDelete(false);
dbBuilder.Entity<Trip>().HasRequired(t => t.Destination).WithRequiredDependent().Map(m => m.MapKey("Trip_Destination")).WillCascadeOnDelete(false);
This is how I use my ApplicationDbContext:
public class TripRepository
private ApplicationDbContext _db;
public TripRepository(ApplicationDbContext context)
this._db = context;
public void AddTrips(List<Trip> trips)
_db.Database.Log = message => Debug.Write(message);
_db.Trips.AddRange(trips);
_db.SaveChanges();
I use my TripRepository like this:
ApplicationDbContext context = HttpContext.GetOwinContext().Get<ApplicationDbContext>();
var tr = new TripRepository(context);
tr.AddTrips(trips); // trips is a valid List<Trip>
The SaveChanges() method of the ApplicationDbContext throws a System.Data.Entity.Core.UpdateException each time I call it, even if I'm sure the trips that I add to the DbSet is a valid List, I checked it with the debugger.
The exception is the only feedback I have, so I really don't know what I'm doing wrong here.. Any ideas?
This is the only feedback I get in the debug output window:
Exception levée : 'System.Data.Entity.Core.UpdateException' dans
EntityFramework.dll
Exception levée : 'System.Data.Entity.Core.UpdateException' dans
EntityFramework.SqlServer.dll
Exception levée : 'System.Data.Entity.Infrastructure.DbUpdateException' dans
EntityFramework.dll
Exception levée : 'System.Data.Entity.Infrastructure.DbUpdateException' dans
System.Web.Mvc.dll
Exception levée : 'System.Data.Entity.Infrastructure.DbUpdateException' dans
System.Web.Mvc.dll
This is how I fill the List that I give to the AddTrips method afterwards (where trip is a viewmodel that I get from a form):
trips.Add(new Trip()
Date = trip.Date,
Price = trip.Price,
Seats = trip.Seats,
Organizer = user,
Departure = new TripCheckpoint() Place = trip.DeparturePlace, Hour = trip.DepartureHour.TimeOfDay ,
Destination = new TripCheckpoint() Place = trip.DestinationPlace, Hour = trip.DestinationHour.TimeOfDay
);
InnerException with details?
– Ben
47 mins ago
I use EF (not EF Core). I have nothing more specific than "System.Data.Entity.Core.UpdateException"
– Warren
39 mins ago
The
Trip
class contains a lot of navigation properties. If all they are disconnected entities, adding trip to the context will mark all them as new, hence the SaveChanges
will try to insert new records, which most likely is not your intent. Btw, the exception either directly or inside inner exception should contain more information what operation failed. Also the Debug window should show the commands.– Ivan Stoev
20 mins ago
Trip
SaveChanges
I don't think my entities are disconnected, I just added to the post how I build those. I have also added the result I get in the output.
– Warren
5 mins ago
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
did u used EF or EF Core?
– ershoaib
51 mins ago