C# — Want to access a class property(field) directly from the object
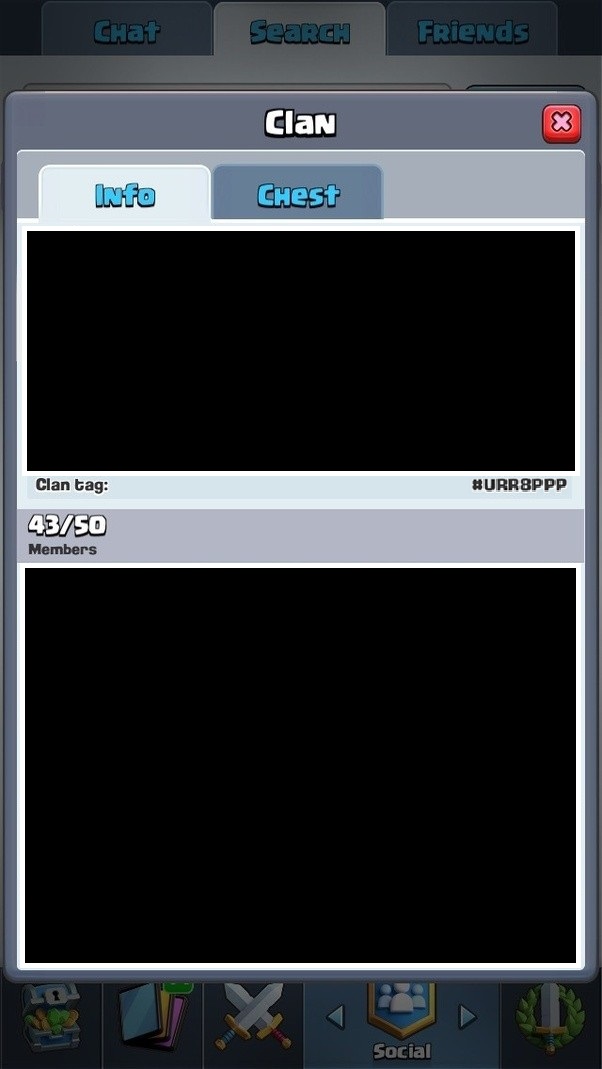

C# — Want to access a class property(field) directly from the object
Okay, I apologise for the awkwardness of the subject here, the very fact that I'm not even sure how to word the question itself properly hasn't helped me in trying to find a solution.
I've written a class that I want to use as a global data interface for interacting with data coming in from a web-based API. As such, the class is able to take in a value of virtually any base-type and work as if it was that base-type.
Under the hood I store the "value" of the object as a string, and, for the most part, the class acts as a clone of the String object, except that it can try to impersonate any other base class in-context.
This is all kind of superfluous though, my problem / question is, how can I make it so that fields of this type are able to interact with it directly instead of through an Accessor?
For Example:
public class PolyVar
protected string _myValue;
public PolyVar() this._myValue = "";
public PolyVar(string value) this._myValue = value;
public string Value get => this._myValue; set => this._myValue = value;
Then, somewhere in the project I WANT do this:
string temp = "";
PolyVar work = ""; // in lieu of "PolyVar work = new PolyVar();"
temp = "Some string data here. " + work; // using just "work" instead of "work.Value"
So can you construct the class in such a way that accessing/modifying
one of its properties (in this case, "_myValue") directly through the class itself is possible in lieu of necessitating the use of an Accessor? (as the base classes already all support?)
Thanks!
sort of defeats the entire purpose of encapsulation. why have a property then, why not just make a public field? i guess you could also make individual methods that modify the field separately, in the way you are looking for, and just call that instead.
– d.moncada
11 mins ago
I'm afraid that's not possible, at least not with the way you currently want it.
– Haytam
11 mins ago
Are you looking for the implicit operator? With that, you can define that other data types (like
string
) can be implicitly converted to your data type, and also the other way around.– Corak
7 mins ago
string
2 Answers
2
Modify your PolyVar
class so that it overrides the ToString()
method:
PolyVar
ToString()
public class PolyVar
protected string _myValue;
public PolyVar() this._myValue = "";
public PolyVar(string value) this._myValue = value;
public string Value get => this._myValue; set => this._myValue = value;
public override string ToString()
return this.Value.ToString();
That allows you to do the following:
PolyVar work = new PolyVar();
string temp = "Some string data here. " + work.ToString();
As your explanation states that you are using multiple objects of PolyVar
I don't really see a way which allows you to skip using the constructor.
PolyVar
... and "adding" the instance to a string automatically calls
.ToString()
. So you can actually write: string temp = "Some string data here. " + work;
– Corak
5 mins ago
.ToString()
string temp = "Some string data here. " + work;
You have to 1) create an implicit conversion from string to PolyVar, and 2) override ToString()
so it is converted properly to a simple string when needed.
ToString()
public class PolyVar
protected string _myValue;
public PolyVar() this._myValue = "";
public PolyVar(string value) this._myValue = value;
public string Value get return this._myValue; set this._myValue = value;
// Override ToString() so "" + polyVar1 is correctly converted.
public override string ToString()
return this.Value;
// Create an implicit cast operator from string to PolyVar
public static implicit operator PolyVar(string x)
return new PolyVar( x );
class Ppal
static void Main()
PolyVar work = "data"; // in lieu of "PolyVar work = new PolyVar();"
string temp = "Some string data here: " + work;
System.Console.WriteLine( temp );
Hope this helps.
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
Wondering why you want such value storage class.
– Ondrej Tucny
18 mins ago