Spring - Hibernate: Unable to Save object data to table with HibernateTemplate
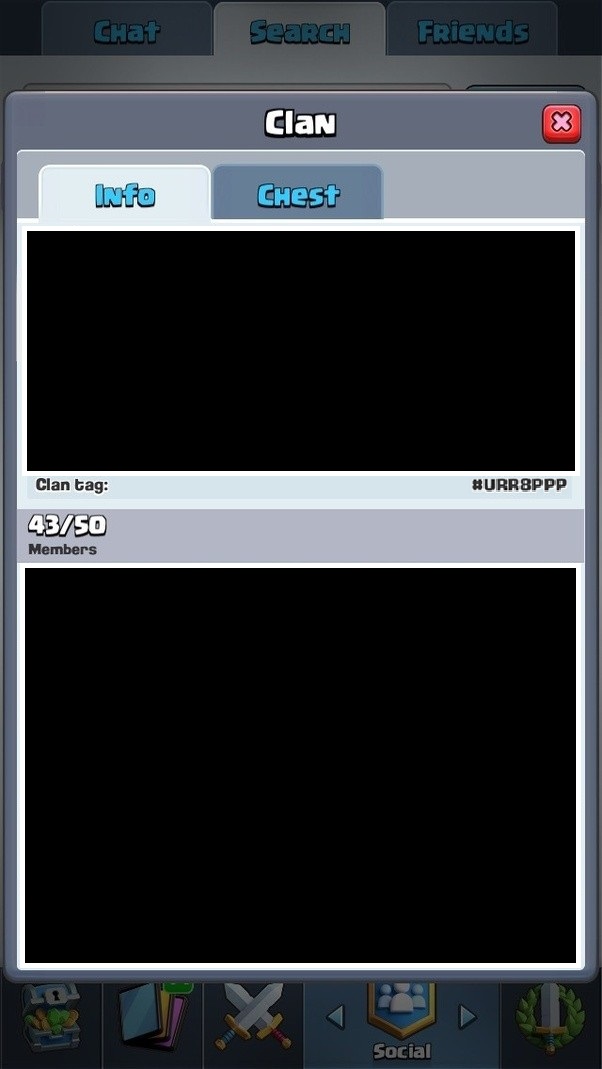

Spring - Hibernate: Unable to Save object data to table with HibernateTemplate
I have looked throughout stackoverflow and I can not seem to find an answer to my query pertaining to saving Created Objects to a Database Table (MySQL) using the HibernateTemplate. I am using Hibernate 5 and the latest iteration of Spring 5.0.7. I am very new to Spring-Hibernate here is the code I have written thus far:
applicationContext.xml:
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context.xsd">
<bean id = "dataSource" class="org.springframework.jdbc.datasource.DriverManagerDataSource">
<property name="driverClassName" value="com.mysql.cj.jdbc.Driver" />
<property name="url" value="jdbc:mysql://localhost:3306/edureka?useLegacyDatetimeCode=false&serverTimezone=Europe/Amsterdam&useSSL=false" />
<property name="username" value="admin" />
<property name="password" value="password" />
</bean>
<bean id= "mySessionFactory" class = "org.springframework.orm.hibernate5.LocalSessionFactoryBean">
<property name="dataSource" ref="dataSource" />
<property name="mappingResources">
<list>
<value>mobilephone.hbm.xml</value>
</list>
</property>
<property name="hibernateProperties">
<props>
<prop key="hibernate.dialect">org.hibernate.dialect.MySQL5Dialect</prop>
<prop key="hibernate.hbm2ddl.auto">update</prop>
<prop key="hibernate.show_sql">true</prop>
</props>
</property>
</bean>
<bean id = "dao" class = "co.module12.MobilePhoneDAO">
<property name="sessionFactory" ref = "mySessionFactory"/>
</bean>
</beans>
Hibernate Mapping File (mobilephone.hbm.xml):
<!DOCTYPE hibernate-mapping PUBLIC
" - //Hibernate/Hibernate Mapping DTD 3.0//EN"
"http://www.hibernate.org/dtd/hibernate-mapping">
<hibernate-mapping>
<class name = "co.module12.MobilePhone" table="mobiles">
<id name="id">
<generator class="increment"></generator>
</id>
<property name="manfacturer" />
<property name="name" />
<property name="price" />
<property name="colour" />
</class>
</hibernate-mapping>
POJO class (Java Bean) MobilePhone.java:
package co.module12;
/**
*
* @author NetBeans
*/
public class MobilePhone
private String manfacturer;
private String colour;
private Double price;
private String name;
private Integer id;
public MobilePhone()
public String getManfacturer()
return manfacturer;
public void setManfacturer(String manfacturer)
this.manfacturer = manfacturer;
public String getColour()
return colour;
public void setColour(String colour)
this.colour = colour;
public Double getPrice()
return price;
public void setPrice(Double price)
this.price = price;
public String getName()
return name;
public void setName(String name)
this.name = name;
public Integer getId()
return id;
public void setId(Integer id)
this.id = id;
MobilePhoneDAO.java:
package co.Module12;
import org.hibernate.SessionFactory;
import org.springframework.orm.hibernate5.HibernateTemplate;
import org.springframework.transaction.annotation.Transactional;
/**
*
* @author NetBeans
*/
public class MobilePhoneDAO
HibernateTemplate template;
SessionFactory factory;
public void setSessionFactory(SessionFactory factory)
template = new HibernateTemplate(factory);
public void saveMobile(MobilePhone phone)
template.setCheckWriteOperations(false);
template.save(phone);
System.out.println(phone.getId());
System.out.println(phone.getManfacturer());
System.out.println(phone.getName());
public void updateMobile(MobilePhone phone)
template.update(phone);
public void deleteMobile(MobilePhone phone)
template.delete(phone);
Main File:
package co.Module12;
import org.springframework.context.support.ClassPathXmlApplicationContext;
/**
*
* @author NetBeans
*/
public class Module12MainClass
public static void main(String args)
ClassPathXmlApplicationContext context = new ClassPathXmlApplicationContext("applicationContext.xml");
MobilePhoneDAO dao = (MobilePhoneDAO)context.getBean("dao");
/*Create Mobile Phone One to Map to mySQL database*/
MobilePhone samsungOne = new MobilePhone();
samsungOne.setColour("black");
samsungOne.setManfacturer("Samsung");
samsungOne.setName("Galaxy S9+");
samsungOne.setPrice(55158.25);
samsungOne.setId(1001);
dao.saveMobile(samsungOne);
/*Create Mobile Phone Two to Map to mySQL database*/
MobilePhone lgPhone = new MobilePhone();
lgPhone.setColour("gray");
lgPhone.setManfacturer("LG");
lgPhone.setName("G7");
lgPhone.setPrice(58782.25);
lgPhone.setId(1002);
dao.saveMobile(lgPhone);
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
Comments
Post a Comment