Write a program that calculates the minimum fixed monthly payment needed in order pay off a credit card balance within 12 months
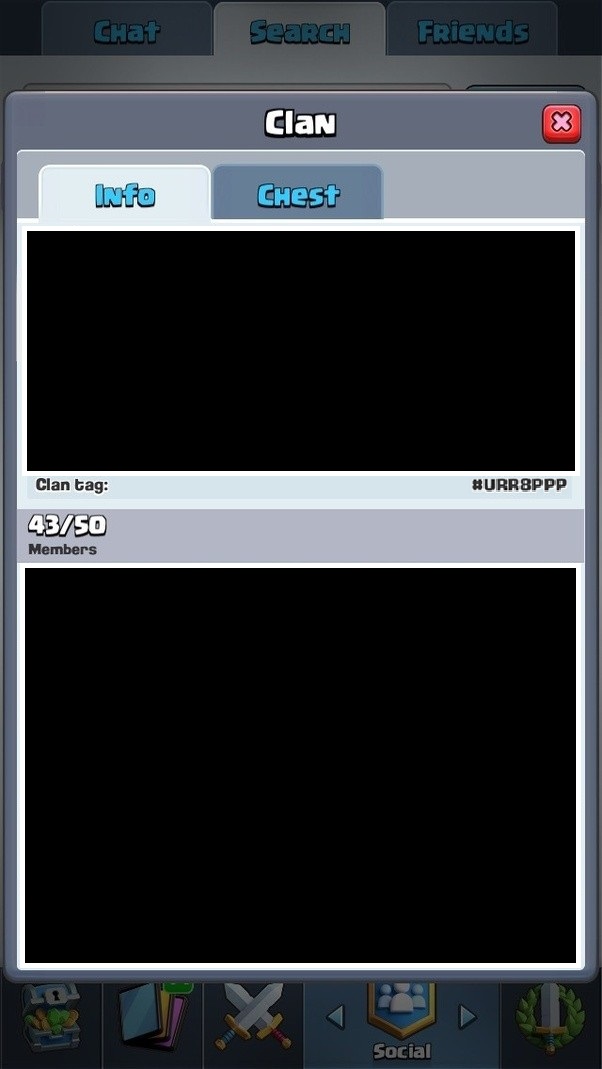

Write a program that calculates the minimum fixed monthly payment needed in order pay off a credit card balance within 12 months
This question is for python 2.7. The question asks to write a program that calculates the minimum fixed monthly payment needed in order pay off a credit card balance within 12 months.By a fixed monthly payment, we mean a single number which does not change each month, but instead is a constant amount that will be paid each month.
In this problem, we will not be dealing with a minimum monthly payment rate.
The following variables contain values as described below:
balance - the outstanding balance on the credit card
annualInterestRate - annual interest rate as a decimal
The program should print out one line: the lowest monthly payment that will pay off all debt in under 1 year.
Assume that the interest is compounded monthly according to the balance at the end of the month (after the payment for that month is made). The monthly payment must be a multiple of $10 and is the same for all months. Notice that it is possible for the balance to become negative using this payment scheme, which is okay. A summary of the required math is found below:
Monthly interest rate = (Annual interest rate) / 12
Updated balance each month = (Previous balance - Minimum monthly payment) x (1 + Monthly interest rate)
I came up with a code for the question; however, I repeatedly got an infinite loop.
b = balance = 3329
air = annualInterestRate = 0.2
monthlyInterestRate = (air/12)
mmp = minimumMonthlyPayment = (balance * monthlyInterestRate)
month = 0
while month <= 12:
b = ((b - mmp) * (1 + (air/12)))
month = 1 + month
if b <= 0 and month == 12:
break
elif b > 0 and month == 12:
b = balance
month = 0
mmp = minimumMonthlyPayment + 10.00
print str('Lowest Payment: ' + str(round(mmp, 2)))
Can someone help me fix this code? For the given balance the lowest payment is 310...I'm not sure how to get this...
9 Answers
9
monthlyInterestRate = annualInterestRate/12
monthlyPayment = 0
newbalance = balance
while newbalance > 0:
monthlyPayment += 10
newbalance = balance
month = 1
while month <= 12 and newbalance > 0:
newbalance -= monthlyPayment
interest = monthlyInterestRate * newbalance
newbalance += interest
month += 1
newbalance = round(newbalance,2)
monthlyPayment = 0
monthlyInterestRate = annualInterestRate /12
newbalance = balance
month = 0
while newbalance > 0:
monthlyPayment += 10
newbalance = balance
for month in range(1,13):
newbalance -= monthlyPayment
newbalance += monthlyInterestRate * newbalance
month += 1
print " Lowest Payment:", monthlyPayment
This code is a bit weird, namely lines like:
mmp = minimumMonthlyPayment = (balance * monthlyInterestRate)
with the double equal signs.
This code doesn't get stuck:
balance = 5000
annualInterestRate = 0.2
monthlyInterestRate = (annualInterestRate/12)
minimumMonthlyPayment = (balance * monthlyInterestRate)
month = 0
while month <= 12:
balance = ((balance - minimumMonthlyPayment) * (1 + monthlyInterestRate))
month = 1 + month
if balance <= 0 and month == 12:
break
elif balance > 0 and month == 12:
month = 0
minimumMonthlyPayment + 1.00
print str('Lowest Payment: ' + str(round(minimumMonthlyPayment, 2)))
But doesn't return the answer you're looking for.
well the teacher puts in a number of things in place of balance and annualInterestRate as long as it checks correctly I'm fine...but the guess and check program (as you can see) isn't really written so well...
– user1721258
Oct 16 '12 at 3:43
This last code (from Dan Steingart) doesn't work either, for example: balance = 4773, annualInterestRate = 0.2. The result gives the value 450, instead of 440!!
– user3362007
Feb 27 '14 at 19:15
First of all you have to fix month <= 12
make it month < 12
secondly you are using a form which is not needed here
balance = ((balance - minimumMonthlyPayment) * (1 + monthlyInterestRate))
because simply you don't have to use the minimum payment here
Your code is too bad
Try this:
balance = 3329
annualInterestRate = 0.2
monthlyPayment = 10
monthlyInterestRate = interestRate/12
newbalance = balance - 10
while newbalance > 0:
monthlyPayment += 10
newbalance = balance
month = 0
while month < 12 and newbalance > 0:
month += 1
interest = monthlyInterestRate * newbalance
newbalance -= monthlyPayment
newbalance += interest
newbalance = round(newbalance,2)
print " Lowest Payment:", monthlyPayment
This should give you the right answer in all of the cases:
monthlyPayment = 10
monthlyInterestRate = annualInterestRate /12
newbalance = balance - 10
while newbalance > 0:
monthlyPayment += 10
newbalance = balance
month = 0
while month < 12 and newbalance > 0:
newbalance -= monthlyPayment
interest = monthlyInterestRate * newbalance
newbalance += interest
month += 1
newbalance = round(newbalance,2)
print " Lowest Payment:", monthlyPayment
balance = 3329
annualInterestRate = 0.2
monthlyInterestRate = annualInterestRate/12
monthlyPayment = 0
newbalance = balance
while newbalance > 0:
monthlyPayment += 10
newbalance = balance
month = 1
while month <= 12 and newbalance > 0:
newbalance -= monthlyPayment
newbalance += (monthlyInterestRate * newbalance)
month += 1
print "Lowest Payment:",monthlyPayment
Think this will be the best solution to your doubt..and satisfies the answer
I think this is a fairly simple way of doing it:
x = 50
o = balance
a = [1,2,3,4,5,6,7,8,9,10,11,12]
monthly = annualInterestRate/12
while balance>0:
for months in a:
u = balance - x
balance = u + (monthly * u)
if balance > 0:
x += 10
else:
break
print x
This is basically a slightly improved version of randomizertech's answer, which allows user to input different variables instead of only doing it for fixed values of Initial balance and Annual interest rate.
And in the end it prints some more variables that would be useful to a user.
InitialBalance = float(raw_input("Enter your current balance: "))
InterestRate = float(raw_input("Enter the yearly interest rate as a decimal: "))
monthlyPayment = 0
monthlyInterestRate = InterestRate/12
balance = InitialBalance
while balance > 0:
monthlyPayment += 10
balance = InitialBalance
numMonths = 0
while numMonths < 12 and balance > 0:
numMonths += 1
interest = monthlyInterestRate * balance
balance -= monthlyPayment
balance += interest
balance = round(balance,2)
print "RESULT"
print "Monthly payment to pay off debt in 1 year: " , monthlyPayment
print "Number of months need to pay the debt: " , numMonths
print "Balance after debt is paid: " , balance
Could you please edit in an explanation of why this code answers the question? Code-only answers are discouraged, because they don't teach the solution.
– Nathan Tuggy
Dec 13 '15 at 1:35
Sorry, noob here. I was actually looking for a solution to a similar problem, where Monthly payment is obtained via bisection instead of being increased by 10. Then I ran into this so I just pasted what I had. Just realized very similar code was already posted too.
– ZeeCpt
Dec 13 '15 at 12:24
for i in range(12):
balance = balance - (balance * monthlyPaymentRate) + ((balance - (balance * monthlyPaymentRate)) * (annualInterestRate/12))
print("Remaining balance:", round(balance, 2))
Correct 10/10
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
This above code will give u correct answer..
– Kamal
Sep 5 '14 at 12:26