Array.includes() to find object in array
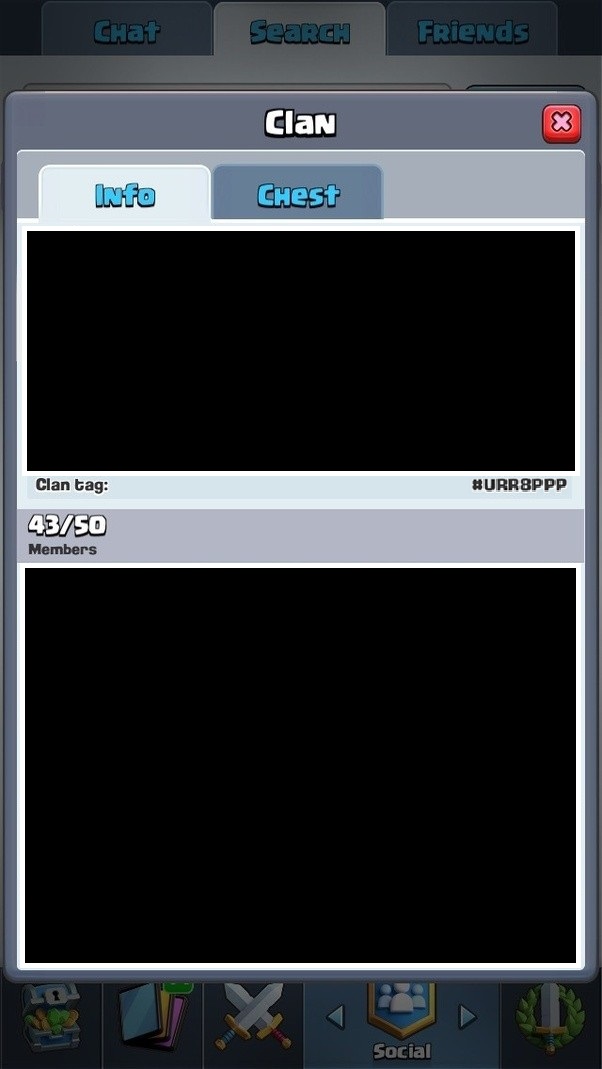

Array.includes() to find object in array
I'm attempting to find an object in an array using Array.prototype.includes
. Is this possible? I realize there is a difference between shallow and deep comparison. Is that the reason the below code returns false?
Array.prototype.includes
I won't be surprised if this question gets downvoted or marked as a duplicate, but I could not find a relevant answer for Array.includes()
. I'll delete if the answer exists elsewhere. Thank you.
Array.includes()
a: 'b' == a: 'b'
false
Yes, you can use Array.prototype.includes to find an object in an array, but it must be the exact same object, not a newly created object like your screenshot shows. This works:
const a = ; console.log( [a].includes( a ) );
.– Paulpro
16 hours ago
const a = ; console.log( [a].includes( a ) );
4 Answers
4
Array.includes
compares by object identity just like obj === obj2
, so sadly this doesn't work unless they are references to the same object. You can often use Array.prototype.some()
instead which takes a function:
Array.includes
obj === obj2
Array.prototype.some()
const arr = [a: 'b']
console.log(arr.some(item => item.a === 'b'))
but of course you then need to write a small function that defines what you mean by equality.
Its' because both of the objects are not the same. Both are stored at different place in memory and the equality operation results false
.
false
But if you search for the same object, then it will return true
.
true
Also, have a look at the below code, where you can understand that two identical objects also results false
with the ===
operator.
false
===
For two objects to return true
in ===
, they should be pointing to same memory location.
true
===
This is because the includes
checks to see if the object is in the array, which it in fact is not:
includes
> a: 'b' === a: 'b'
false
This is because the test for equality is not testing if the objects are the same, but whether the point to the same object. Which they don't.
You are on the right track but, the issue is the difference between reference and value types, you currently are using a reference type (object literal), so when you compare what is in the array with what you have, it will compare the references and not the values. this is what I mean:
var ar = ;
var x = a: "b", c: "d" ;
ar.push(x);
// this will log true because its the same reference
console.log("should be true: ", ar[0] === x);
ar.push(a: "b", c: "d" );
// this will log false because i have created
// a different reference for a new object.
console.log("should be false: ", ar[1] === x);
// Think of it like this
var obja = foo: "bar" ; // new reference to 'obja'
var objb = foo: "bar" ; // new reference to 'objb'
var valuea = 23;
var valueb = 23;
// 'obja' and 'obja' are different references
// although they contain same property & value
// so a test for equality will return false
console.log("should be false: ", obja === objb);
// on the other hand 'valuea' and 'valueb' are
// both value types, so an equality test will be true
console.log("should be true: ", valuea === valueb);
to achieve what you want, you either have to have added the actual reference, as I did above, or loop through the array and compare by unique property of the objects.
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
because
a: 'b' == a: 'b'
isfalse
?– Federkun
16 hours ago