How to get values from input types using this.refs in reactjs?
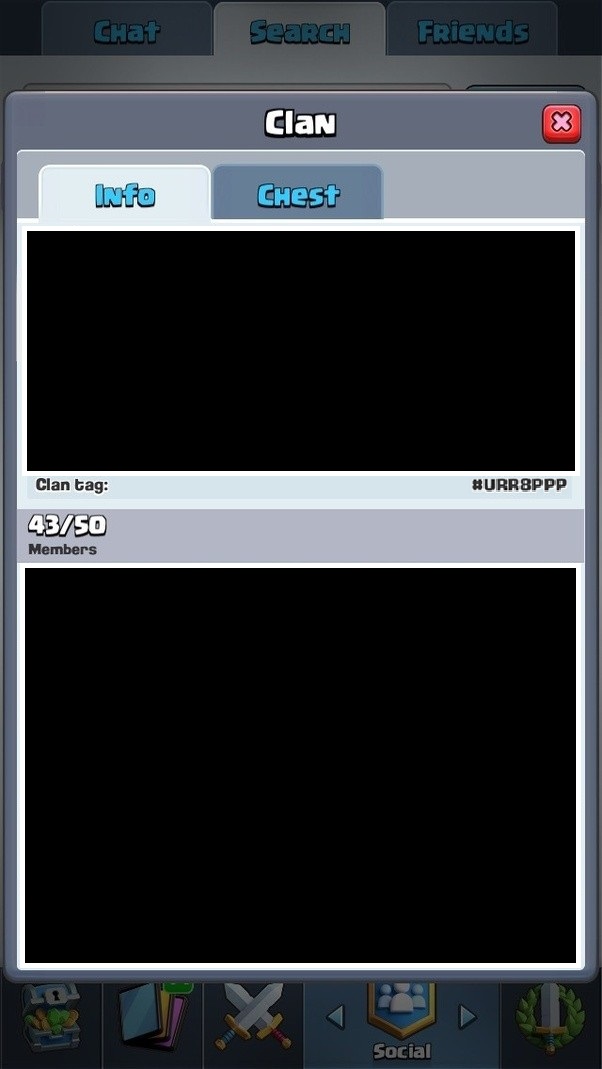

How to get values from input types using this.refs in reactjs?
Not able to get values of input type using this.refs...
how to get that values from input type
export class BusinessDetailsForm extends Component
submitForm(data)
console.log(this.refs.googleInput.value)
reder()
return(
<form onSubmit=this.submitForm>
<Field type="text"
name="location"
component=GoogleAutoComplete
id="addressSearchBoxField"
ref="googleInput"
/>
</form>
)
}
4 Answers
4
You should avoid ref="googleInput"
as it is now considered legacy. You should instead declare
ref="googleInput"
ref=(googleInput) => this.googleInput = googleInput
Inside of your handler, you can use this.googleInput
to reference the element.
this.googleInput
Then inside of your submitForm
function, you can obtain the text value withthis.googleInput._getText()
submitForm
this.googleInput._getText()
String refs are legacy
https://facebook.github.io/react/docs/refs-and-the-dom.html
If you worked with React before, you might be familiar with an older API where the ref attribute is a string, like "textInput", and the DOM node is accessed as this.refs.textInput. We advise against it because string refs have some issues, are considered legacy, and are likely to be removed in one of the future releases. If you're currently using this.refs.textInput to access refs, we recommend the callback pattern instead.
ref
This doesn't work. I'm getting an error saying
Uncaught TypeError: _this.googleInput._getText is not a function
– Thidasa Paranavitharana
Jun 17 at 12:29
Uncaught TypeError: _this.googleInput._getText is not a function
getValue: function()
return this.refs.googleInput.value;
I think the more idiomatic way is to use state
instead of refs
, although it's a little more code in this case since you only have a single input.
state
refs
export class BusinessDetailsForm extends Component
constructor(props)
super(props);
this.state = googleInput: '' ;
this.defaultValue = 'someValue';
this.handleChange = this.handleChange.bind(this);
this.submitForm = this.submitForm.bind(this);
handleChange(e)
const field, value = e.target;
this.setState( [field]: value );
submitForm()
console.log(this.state.googleInput);
render()
return (
<Formsy.Form onSubmit=this.submitForm id="form_validation">
<Field type="text"
name="googleInput"
onChange=this.handleChange
component=GoogleAutoComplete
floatingLabelText="location"
hintText="location"
id="addressSearchBoxField"
defaultValue=this.defaultValue
onSelectPlace=this.handlePlaceChanged
validate=[ required ]
/>
</Formsy.Form>
);
See https://facebook.github.io/react/docs/forms.html#controlled-components.
In 2018 Refs should be like this:
https://reactjs.org/docs/uncontrolled-components.html
1) In constructor of class you should add something like this.input = React.createRef()
this.input = React.createRef()
2) <input type="text" ref=this.input />
<input type="text" ref=this.input />
3) Get the value: this.input.current.value
this.input.current.value
Full Example:
class NameForm extends React.Component
constructor(props)
super(props);
this.handleSubmit = this.handleSubmit.bind(this);
this.input = React.createRef();
handleSubmit(event)
alert('A name was submitted: ' + this.input.current.value);
event.preventDefault();
render()
return (
<form onSubmit=this.handleSubmit>
<label>
Name:
<input type="text" ref=this.input />
</label>
<input type="submit" value="Submit" />
</form>
);
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
@ShubhamKhatri Please consider downvoting if you feel this answer does not contribute to the question. The OP asked how to use
ref
to obtain the value of an input field, this answer provides a solution to help satisfy that.– Dan
Mar 31 '17 at 10:03