Box2d Rotate body around point using forces or impulses [on hold]
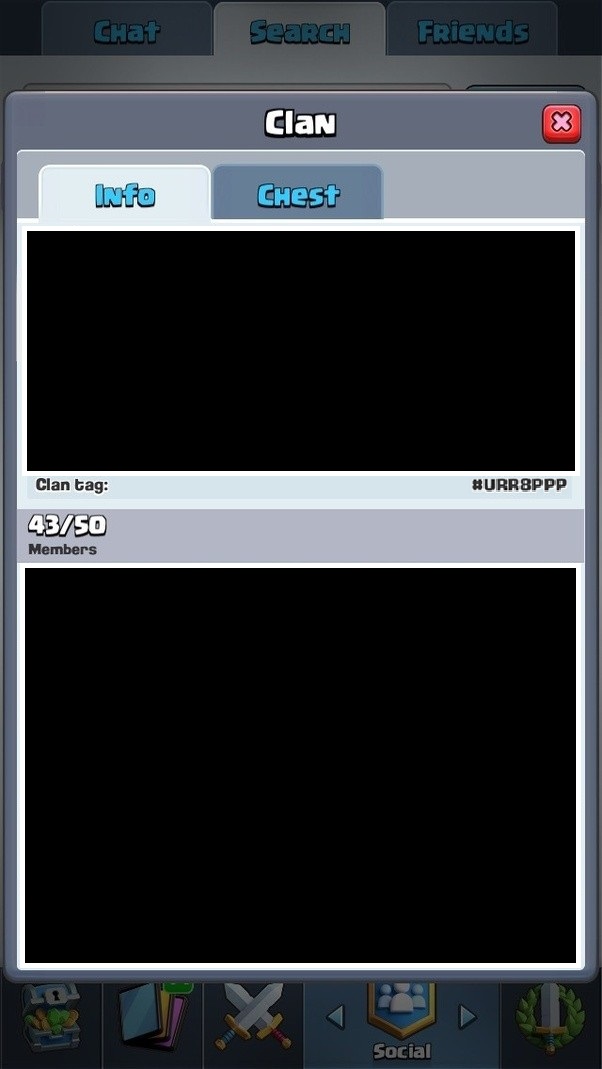

Box2d Rotate body around point using forces or impulses [on hold]
I have a body that should move around some point. If I press "right" body moves clockwise, if I press left body moves counterclockwise direction. Which force I should apply to do that?
Please edit the question to limit it to a specific problem with enough detail to identify an adequate answer. Avoid asking multiple distinct questions at once. See the How to Ask page for help clarifying this question. If this question can be reworded to fit the rules in the help center, please edit the question.
2 Answers
2
I think you need to create a static body at the point you wish to rotate around and then connect your rotating body and it using a b2DisanceJoint. From the Box2d Manual:
b2DistanceJointDef jointDef;
jointDef.Initialize(myBodyA, myBodyB, worldAnchorOnBodyA, worldAnchorOnBodyB); jointDef.collideConnected = true;
world->CreateJoint(&jointDef);
Then you will have to apply some force to your body (let's say it was myBodyB) to get it moving in the right direction so it rotates around myBodyA. This is going to require some math.
What you want to do is calculate the vector that points perpendicular to the vector pointing from myBodyB to myBodyA. You can do this by finding the vector from myBodyB to myBodyA, normalizing it, taking the skew of it (rotate by PI/2), and then using it as a force direction. Something like:
// Calculate Tangent Vector
b2Vec2 radius = myBodyB.GetPosition()-myBodyA.GetPosition();
b2Vec2 tangent = radius.Skew();
tangent.Normalize();
// Apply some force along tangent
myBodyB.ApplyForceToCenter(body->GetMass()*acceleration*vTangent);
If you read this correctly, you can see F = m * a * tangentVector; that is to say, you are applying force in the direction of the tangent. I believe it will rotate it clockwise (if I did the math right). Regardless, a negative on the force will move it in the opposite direction.
To stop the body rotating, you can use the SetLinearDamping(dampingValue) on it. So as long as you apply force, it will continue to rotate. If you stop applying force, it should gently stop. You can control the speed up/slow down by the acceleration value and the dampingValue parameters.
If you really want good control over the speed, I believe you can do this to clamp the speed:
b2Vec2 linVel = myBodyB.GetLinearVelocity();
linVel.Normalize();
linVel *= maxSpeed;
myBodyB.SetLinearVelocity(linVel);
Was this helpful?
You'd use either
body->ApplyAngularImpulse(2.0);
or
body->ApplyTorque(2.0);
Use a positive force for rotating clockwise, and a negative force for rotating counterclockwise.
In that case you'll have to set up another body (probably a static body) as an anchor point and connect them with a revolute joint. The location of the revolute joint will be the axis of rotation.
– Mike
Nov 8 '13 at 17:51
How about using a joint? Otherwise, you will need to simultaneously move and rotate the body, and calculate the necessary movement yourself.
– iforce2d
Nov 8 '13 at 17:58
No. That will make body rotate body around it`s mass center. I need to rotate around some point of the world.
– Sergei Alekseev
Nov 8 '13 at 13:08