Fading background image with javascript on image switch
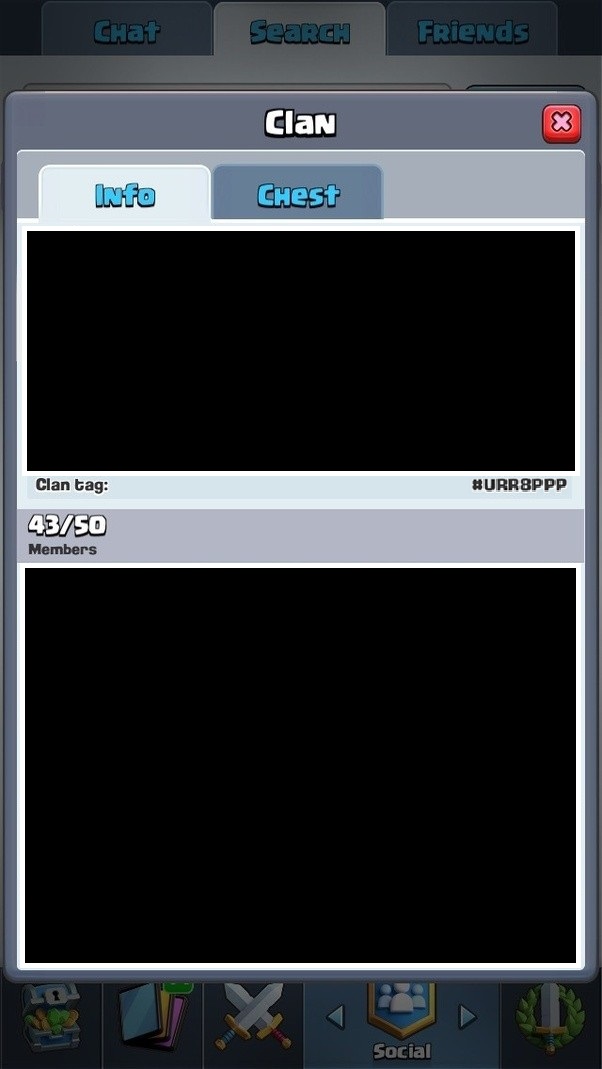

Fading background image with javascript on image switch
I have three images which my two div's switch between. However, the animation appears too rough. How do I apply a fade right before the switch?
<div class="my-images">
<div id="image-1"></div>
<div id="image-2"></div>
</div>
<script>
function displayNextImage()
x = (x === images.length - 1) ? 0 : x + 1;
document.getElementById("image-1").style.backgroundImage = images[x];
document.getElementById("image-2").style.backgroundImage = images[x];
function startTimer()
setInterval(displayNextImage, 10000);
var images = ,
x = 0;
images[0] = "url('images/snow.jpeg')";
images[1] = "url('images/nike.jpeg')";
images[2] = "url('images/motor.jpeg')";
</script>
This loops continuously so I do not want it just fading in on the first load.
2 Answers
2
Without JQuery you'll have cross-browser compatibility issue.
So i suggest you to use JQuery to achieve this.
<div class="my-images">
<div class="my-image" id="image-1"></div>
<div class="my-image" id="image-2"></div>
</div>
<script>
function displayNextImage()
$("#image-" + x).css('backgroundImage', images[x]).show('slow');
x++;
var images = ,
x = 0;
images[0] = "url('images/snow.jpeg')";
images[1] = "url('images/nike.jpeg')";
images[2] = "url('images/motor.jpeg')";
</script>
And you have to add this to css:
.my-image
display:none;
In case you not use display: block
to you element:
Your CSS will be:
display: block
.my-image
display:whatYouWant;
Then need add the document ready()
function and change show()
to fadeIn()
:
ready()
show()
fadeIn()
$(document).ready(function()
$(".my-image").hide();
);
function displayNextImage()
$("#image-" + x).css('backgroundImage', images[x]).fadeIn('slow');
x++;
This will work because fadeIn()
set to display the previous value.
fadeIn()
If you want div visible before image adding, remove $(document).ready()
call and edit displayNextImage()
:
$(document).ready()
displayNextImage()
function displayNextImage()
$("#image-" + x).hide().css('backgroundImage', images[x]).fadeIn('slow');
x++;
check edited answer
– Frighi
9 hours ago
On load the div is still missing and this only fades slow the first time after that it doesn't anymore then it just changes the pictures. Thanks for the help anyway.
– James
9 hours ago
Ops, I didn't understand you want visible divs before images, I edited answer again
– Frighi
9 hours ago
OMG I love you thank you.
– James
8 hours ago
You can do it with CSS animation, for each cycle:
1) swap the image sources like you are already doing
2) reset the fade in animation, more info here
3) increment your index
const imgs = [
'https://source.unsplash.com/iMdsjoiftZo/100x100',
'https://source.unsplash.com/ifhgv-c6QiY/100x100',
'https://source.unsplash.com/w5e288LU8SU/100x100'
];
let index = 0;
const oldImage1 = document.getElementById('oldImage1');
const newImage1 = document.getElementById('newImage1');
const oldImage2 = document.getElementById('oldImage2');
const newImage2 = document.getElementById('newImage2');
window.setInterval(() =>
// put new image in old image
oldImage1.src = newImage1.src;
oldImage2.src = newImage2.src;
// Set new image
newImage1.src = imgs[index];
newImage2.src = imgs[index];
// reset animation
newImage1.style.animation = 'none';
newImage1.offsetHeight; /* trigger reflow */
newImage1.style.animation = null;
newImage2.style.animation = 'none';
newImage2.offsetHeight; /* trigger reflow */
newImage2.style.animation = null;
// increment x
index = index + 1 >= imgs.length ? 0 : index + 1;
, 1500);
.parent
position: relative;
top: 0;
left: 0;
float: left;
.oldImage1
position: relative;
top: 0;
left: 0;
.newImage1
position: absolute;
top: 0;
left: 0;
.oldImage2
position: relative;
top: 0;
left: 100;
.newImage2
position: absolute;
top: 0;
left: 100;
.fade-in
animation: fadein 1s;
@keyframes fadein
from opacity: 0;
to opacity: 1;
<div class="parent">
<img id="oldImage1" class="oldImage1" src="https://source.unsplash.com/ifhgv-c6QiY/100x100" />
<img id="newImage1" class="newImage1 fade-in" src="https://source.unsplash.com/w5e288LU8SU/100x100" />
</div>
<div class="parent">
<img id="oldImage2" class="oldImage2" src="https://source.unsplash.com/ifhgv-c6QiY/100x100" />
<img id="newImage2" class="newImage2 fade-in" src="https://source.unsplash.com/w5e288LU8SU/100x100" />
</div>
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
I understand what you did but on load it makes the divs dissapear then reappear after the timer with the new image. I do not want the divs to dissapear on load.
– James
9 hours ago